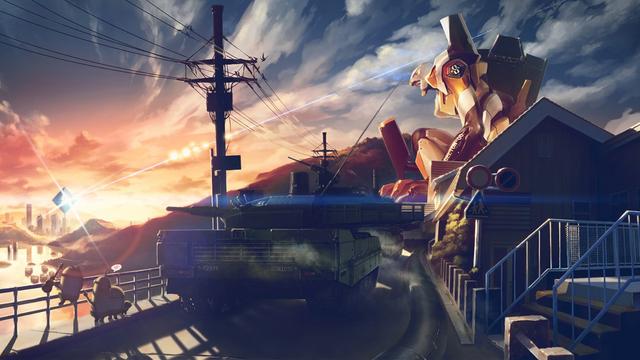
What is Django? What are its advantages? Link to What is Django? What are its advantages?
Django is a high-level Python web framework designed for rapid development of secure and maintainable web applications.
It follows the principles of “Don’t Repeat Yourself” (DRY) and “Convention Over Configuration”.
Advantages:
- Rapid Development: Django’s design enables developers to build and deploy applications quickly.
- Built-in Features: Provides out-of-the-box functionality like authentication systems, ORM, form handling, and more.
- Security: Includes default protections against common security threats such as SQL injection, cross-site request forgery (CSRF), and cross-site scripting (XSS).
- Strong Community Support: Backed by an active developer community with extensive third-party packages and extensions.
Explain Django’s MVT Pattern Link to Explain Django’s MVT Pattern
The MVT (Model-View-Template) pattern is Django’s architectural design:
- Model: Handles database interactions and defines data structures (e.g., table schemas).
- View: Implements business logic, processes requests, interacts with models, and renders templates.
- Template: Renders the user interface using Django’s template language to generate dynamic HTML.
How does Django’s ORM work? Link to How does Django’s ORM work?
Django’s ORM (Object-Relational Mapping) allows developers to interact with databases using Python objects instead of writing SQL.
Model classes define database table structures, and instances of these classes interact with the database.
Example:
1234
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=100)
birth_date = models.DateField()
What is Middleware in Django? Link to What is Middleware in Django?
Middleware is a layer of components that processes requests and responses globally in Django.
They execute before a request reaches a view or after a response is sent to the client.
Common Middleware:
AuthenticationMiddleware
: Associates users with requests.SessionMiddleware
: Manages session data.CSRFViewMiddleware
: Protects against cross-site request forgery.
How to use Django’s Admin interface? Link to How to use Django’s Admin interface?
Django provides an auto-generated Admin interface for managing data models.
Steps to enable:
- Register models in
admin.py
:
123
from django.contrib import admin
from .models import Author
admin.site.register(Author)
- Access
/admin/
, log in with a superuser account, and manage data.
How to create and apply database migrations in Django? Link to How to create and apply database migrations in Django?
Django uses migrations to manage database schema changes.
Steps:
- Create migrations:
python manage.py makemigrations
- Apply migrations:
python manage.py migrate
How does Django’s URL routing work? Link to How does Django’s URL routing work?
Django’s URL routing maps URL patterns to view functions.
Example:
12345
from django.urls import path
from . import views
urlpatterns = [
path('home/', views.home_view, name='home'),
]
How to handle static and media files in Django? Link to How to handle static and media files in Django?
- Static files (CSS, JS, images): Configure using
STATICFILES_DIRS
andSTATIC_ROOT
. - Media files (user-uploaded content): Configure using
MEDIA_URL
andMEDIA_ROOT
.
What are signals in Django? How to use them? Link to What are signals in Django? How to use them?
Signals allow decoupled components to trigger actions when specific events occur.
Common signals:
pre_save
/post_save
: Triggered before/after a model instance is saved.pre_delete
/post_delete
: Triggered before/after a model instance is deleted.
Example:
1234567
from django.db.models.signals import post_save
from django.dispatch import receiver
from .models import UserProfile
@receiver(post_save, sender=User)
def create_user_profile(sender, instance, created, **kwargs):
if created:
UserProfile.objects.create(user=instance)
Differences between ForeignKey, OneToOneField, and ManyToManyField Link to Differences between ForeignKey, OneToOneField, and ManyToManyField
- ForeignKey: A many-to-one relationship (e.g., a book belongs to one author).
- OneToOneField: A one-to-one relationship (e.g., a user has one profile).
- ManyToManyField: A many-to-many relationship (e.g., students and courses).
How to optimize Django query performance? Link to How to optimize Django query performance?
Optimization techniques:
- Use
select_related()
andprefetch_related()
for related queries. - Use
only()
anddefer()
to load specific fields. - Add database indexes to frequently queried fields.
How to implement permissions in Django? Link to How to implement permissions in Django?
Django’s built-in permission system assigns permissions to users or groups.
Example:
123456789
from django.contrib.auth.models import User, Permission
# Assign permission
user = User.objects.get(username='john')
permission = Permission.objects.get(codename='can_edit')
user.user_permissions.add(permission)
# Check permission
if user.has_perm('app_name.can_edit'):
# User has permission
How does Django handle forms? Link to How does Django handle forms?
Django’s forms
module validates and processes form data using form classes.
Example:
123456789101112
from django import forms
class ContactForm(forms.Form):
name = forms.CharField(max_length=100)
email = forms.EmailField()
# In a view
def contact_view(request):
if request.method == 'POST':
form = ContactForm(request.POST)
if form.is_valid():
# Process data
pass
How to implement caching in Django? Link to How to implement caching in Django?
Django supports multiple caching backends (e.g., memory, file, database).
Configuration example:
12345
CACHES = {
'default': {
'BACKEND': 'django.core.cache.backends.memcached.MemcachedCache',
'LOCATION': '127.0.0.1:11211',
}
}
Usage:
1234
from django.views.decorators.cache import cache_page
@cache_page(60 * 15) # Cache view for 15 minutes
def my_view(request):
...
How to handle async tasks in Django? Link to How to handle async tasks in Django?
Django typically integrates with Celery for asynchronous tasks.
Steps:
- Install Celery:
pip install celery
- Configure Celery (
celery.py
):
1
from celery import Celery
app = Celery('project_name', broker='redis://localhost:6379/0')
- Define tasks:
1234
from celery import shared_task
@shared_task
def my_task():
...
How to build a REST API in Django? Link to How to build a REST API in Django?
Use Django REST Framework (DRF).
Key components:
- Serializer: Handles data serialization/deserialization.
- ViewSet: Implements API logic.
- Router: Auto-generates URL routes.
Example:
12345678910
from rest_framework import serializers, viewsets
from .models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = '__all__'
class MyModelViewSet(viewsets.ModelViewSet):
queryset = MyModel.objects.all()
serializer_class = MyModelSerializer
How to write unit tests in Django? Link to How to write unit tests in Django?
Django’s built-in test framework extends Python’s unittest
.
Example:
123456789
from django.test import TestCase
from .models import MyModel
class MyModelTestCase(TestCase):
def setUp(self):
MyModel.objects.create(name='Test')
def test_model_creation(self):
obj = MyModel.objects.get(name='Test')
self.assertEqual(obj.name, 'Test')